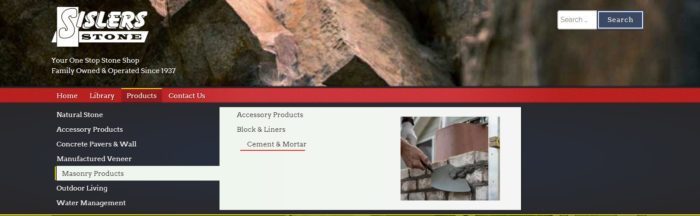
I recently had a need to create a custom, three level navigation in WordPress. The navigation is designed to work with WooCommerce, pulling a product category image from the associated category menu item, and displaying it when the user hovers over the third-level category.
Walker Classes are a well-documented way to customize your navigations within WordPress. You can easily create a three-tiered navigation menu by expanding on the example Walker Class provided in WordPress’ Codex.
class Sislers_Walker extends Walker_Nav_Menu
{
// add classes to ul sub-menus
function start_lvl( &$output, $depth ) {
// depth dependent classes
$indent = ( $depth > 0 ? str_repeat( "\t", $depth ) : '' ); // code indent
$display_depth = ( $depth + 1); // because it counts the first submenu as 0
$classes = array(
'sub-menu',
( $display_depth % 2 ? 'menu-odd' : 'menu-even' ),
( $display_depth >=2 ? 'sub-sub-menu' : '' ),
'menu-depth-' . $display_depth
);
$class_names = implode( ' ', $classes );
// build html
$output .= "\n" . $indent . '<ul class="' . $class_names . '">' . "\n";
$output .= $indent . '<div class="link-wrap">' . "\n";
}
function end_lvl(&$output, $depth = 0, $args = array())
{
$indent = ( $depth > 0 ? str_repeat( "\t", $depth ) : '' ); // code indent
$output .= $indent . '</div>' . "\n" . '</ul>' . "\n";
}
// add main/sub classes to li's and links
function start_el( &$output, $item, $depth, $args ) {
global $wp_query;
$indent = ( $depth > 0 ? str_repeat( "\t", $depth ) : '' ); // code indent
// depth dependent classes
$depth_classes = array(
( $depth == 0 ? 'main-menu-item' : 'sub-menu-item' ),
( $depth >=2 ? 'sub-sub-menu-item' : '' ),
( $depth % 2 ? 'menu-item-odd' : 'menu-item-even' ),
'menu-item-depth-' . $depth
);
$depth_class_names = esc_attr( implode( ' ', $depth_classes ) );
// passed classes
$classes = empty( $item->classes ) ? array() : (array) $item->classes;
$class_names = esc_attr( implode( ' ', apply_filters( 'nav_menu_css_class', array_filter( $classes ), $item ) ) );
// build html
$output .= $indent . '<li id="nav-menu-item-'. $item->ID . '" class="' . $depth_class_names . ' ' . $class_names . '">';
//display featured image
//$output .= $thumbnail;
// link attributes
$attributes = ! empty( $item->attr_title ) ? ' title="' . esc_attr( $item->attr_title ) .'"' : '';
$attributes .= ! empty( $item->target ) ? ' target="' . esc_attr( $item->target ) .'"' : '';
$attributes .= ! empty( $item->xfn ) ? ' rel="' . esc_attr( $item->xfn ) .'"' : '';
$attributes .= ! empty( $item->url ) ? ' href="' . esc_attr( $item->url ) .'"' : '';
$attributes .= ' class="menu-link ' . ( $depth > 0 ? 'sub-menu-link' : 'main-menu-link' ) . '"';
$item_output = sprintf( '%1$s<a%2$s>%3$s%4$s%5$s%6$s</a>%7$s',
$args->before,
$attributes,
$thumbnail,
$args->link_before,
apply_filters( 'the_title', $item->title, $item->ID ),
$args->link_after,
$args->after
);
// build html
$output .= apply_filters( 'walker_nav_menu_start_el', $item_output, $item, $depth, $args );
}
}
The above can be modified very simply to get the thumbnail image associated with the WooCommerce category. In my case, I wanted the category images to appear at the third depth of category menus. This is achievable simply with the below, just above the “//passed classes” line:
// get featured image
if ($depth == 2){
$thumbnail_id = get_woocommerce_term_meta( $item->object_id, 'thumbnail_id', true );
$image_url = wp_get_attachment_image_src( $thumbnail_id, 'medium' )['0'];
$thumbnail = '<div class="nav-thumb"><img src="' . $image_url . '" class="" title="' . $title . '" /></div>';
}
else {
$thumbnail = null;
}
Of course, in both examples, you can customize the HTML output to whatever works for you and your styles. The final bit of code is below:
class Sislers_Walker extends Walker_Nav_Menu
{
// add classes to ul sub-menus
function start_lvl( &$output, $depth ) {
// depth dependent classes
$indent = ( $depth > 0 ? str_repeat( "\t", $depth ) : '' ); // code indent
$display_depth = ( $depth + 1); // because it counts the first submenu as 0
$classes = array(
'sub-menu',
( $display_depth % 2 ? 'menu-odd' : 'menu-even' ),
( $display_depth >=2 ? 'sub-sub-menu' : '' ),
'menu-depth-' . $display_depth
);
$class_names = implode( ' ', $classes );
// build html
$output .= "\n" . $indent . '<ul class="' . $class_names . '">' . "\n";
$output .= $indent . '<div class="link-wrap">' . "\n";
}
function end_lvl(&$output, $depth = 0, $args = array())
{
$indent = ( $depth > 0 ? str_repeat( "\t", $depth ) : '' ); // code indent
$output .= $indent . '</div>' . "\n" . '</ul>' . "\n";
}
// add main/sub classes to li's and links
function start_el( &$output, $item, $depth, $args ) {
global $wp_query;
$indent = ( $depth > 0 ? str_repeat( "\t", $depth ) : '' ); // code indent
// depth dependent classes
$depth_classes = array(
( $depth == 0 ? 'main-menu-item' : 'sub-menu-item' ),
( $depth >=2 ? 'sub-sub-menu-item' : '' ),
( $depth % 2 ? 'menu-item-odd' : 'menu-item-even' ),
'menu-item-depth-' . $depth
);
$depth_class_names = esc_attr( implode( ' ', $depth_classes ) );
// get featured image
if ($depth == 2){
$thumbnail_id = get_woocommerce_term_meta( $item->object_id, 'thumbnail_id', true );
$image_url = wp_get_attachment_image_src( $thumbnail_id, 'medium' )['0'];
$thumbnail = '<div class="nav-thumb"><img src="' . $image_url . '" class="" title="' . $title . '" /></div>';
}
else {
$thumbnail = null;
}
// passed classes
$classes = empty( $item->classes ) ? array() : (array) $item->classes;
$class_names = esc_attr( implode( ' ', apply_filters( 'nav_menu_css_class', array_filter( $classes ), $item ) ) );
// build html
$output .= $indent . '<li id="nav-menu-item-'. $item->ID . '" class="' . $depth_class_names . ' ' . $class_names . '">';
//display featured image
//$output .= $thumbnail;
// link attributes
$attributes = ! empty( $item->attr_title ) ? ' title="' . esc_attr( $item->attr_title ) .'"' : '';
$attributes .= ! empty( $item->target ) ? ' target="' . esc_attr( $item->target ) .'"' : '';
$attributes .= ! empty( $item->xfn ) ? ' rel="' . esc_attr( $item->xfn ) .'"' : '';
$attributes .= ! empty( $item->url ) ? ' href="' . esc_attr( $item->url ) .'"' : '';
$attributes .= ' class="menu-link ' . ( $depth > 0 ? 'sub-menu-link' : 'main-menu-link' ) . '"';
$item_output = sprintf( '%1$s<a%2$s>%3$s%4$s%5$s%6$s</a>%7$s',
$args->before,
$attributes,
$thumbnail,
$args->link_before,
apply_filters( 'the_title', $item->title, $item->ID ),
$args->link_after,
$args->after
);
// build html
$output .= apply_filters( 'walker_nav_menu_start_el', $item_output, $item, $depth, $args );
}
}